UI Test란?
UI Test는 User Interface Testing의 약자로 말 그대로 사용자에게 보여지는 화면이 의도한 방향대로 작업흐름이 올바른지 테스트하는 것이다. xCode에서 UI 테스트는 쿼리로 앱의 UI 개체를 찾고 이벤트를 활성화한 다음 해당 개체에 이벤트를 보내는 방식으로 작동한다. API를 사용하면 UI 개체의 속성 및 상태를 검사하여 예상 상태와 비교할 수 있다.
UI Test 추가방법
xCode 사이드바에 “Show the test navigator” 버튼을 클릭하면 UI 및 Unit 테스트 목록들이 나타나는 걸 알 수 있는데 하단에 “+” 버튼을 통해 새롭게 추가할 수 있다.
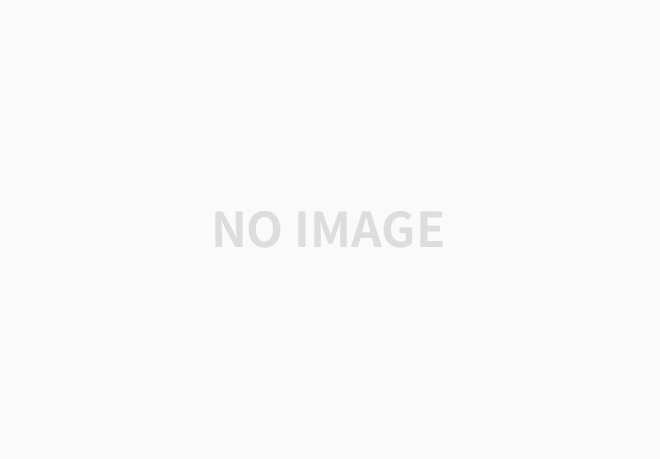
UI Test 예제
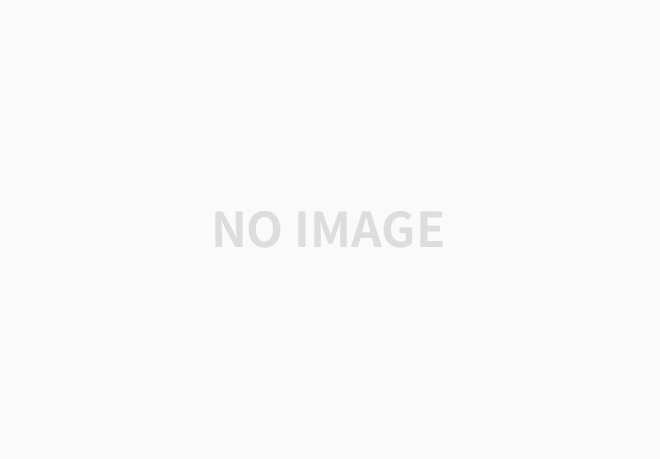
- 테스트할 어플의 오른쪽 하단을 보면 “Slide”와 “Type” 슬라이더 바가 있는데 이것을 누르게 되면 상단에 Label의 test가 바뀌게 설정하였고, 해당 UI 이벤트가 잘 발생하는지 테스트할 예정이다.
전체코드
import XCTest
class UnitTestExampleUITests: XCTestCase {
var app:XCUIApplication!
override func setUpWithError() throws {
try super.setUpWithError()
continueAfterFailure = false
app = XCUIApplication()
app.launch()
}
func testGameStyleSwitch() throws {
// given
let slideButton = app.segmentedControls.buttons["Slide"]
let typeButton = app.segmentedControls.buttons["Type"]
let slideLabel = app.staticTexts["Get as close as you can to: "]
let typeLabel = app.staticTexts["Guess where the slider is: "]
// then
if slideButton.isSelected {
XCTAssertTrue(slideLabel.exists)
XCTAssertFalse(typeLabel.exists)
typeButton.tap()
XCTAssertTrue(typeLabel.exists)
XCTAssertFalse(slideLabel.exists)
} else if typeButton.isSelected {
XCTAssertTrue(typeLabel.exists)
XCTAssertFalse(slideLabel.exists)
slideButton.tap()
XCTAssertTrue(slideLabel.exists)
XCTAssertFalse(typeLabel.exists)
}
}
}
이번에는 mock 객체를 XCUIApplication 클래스 타입으로 선언하였다. XCUIApplication은 테스트 애플리케이션을 시작, 모니터링 및 종료할 수 있는 프록시이다. 내장되어 있는 launch() 함수를 통해 테스트 응용프로그램을 실행할 수 있다. setUpWithError()안에 continueAfterFailure는 테스트를 할 때 실패가 발생하면 테스트 메서드를 계속 실행해야 하는지 여부를 나타내는 Bool Type의 값이다. true이면 테스트가 실패해도 남은 테스트를 마저 실행하고 false이면 실패가 발생하는 즉시 종료된다.
XCUIApplication
XCUIApplication에는 segmentedControls, staticTexts, buttons 등 다양한 UI Components를 담는 딕셔너리가 존재한다. 해당 딕셔너리의 키값은 label의 텍스트 값이나 버튼의 title로 확인이 가능하다. 이 테스트에서는 given 주석 아래에서 “Slide”와 “Type” 란 타이틀을 가진 segmentedControl 컴포넌트를 선언했고, 거기에 맞는 레이블들을 추가로 선언하였다.
then 주석에서는 해당 segemtedControl 버튼들을 클릭하면 해당 label이 존재하는지 안하는지 XCTAssert 함수들을 통해서 유무를 확인하고 테스트 결과를 배출한다.
결과 화면
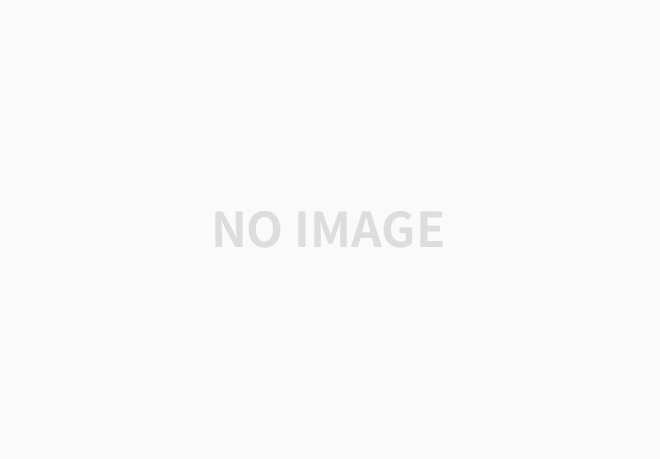
'Swift' 카테고리의 다른 글
[Swift] Privacy Info (0) | 2024.08.18 |
---|---|
<Value Type> Set (1) | 2024.03.03 |
13. Unit Test(3) (0) | 2022.12.23 |
12. Unit Test(2) (0) | 2022.12.16 |
11. Unit Test(1) (0) | 2022.12.09 |